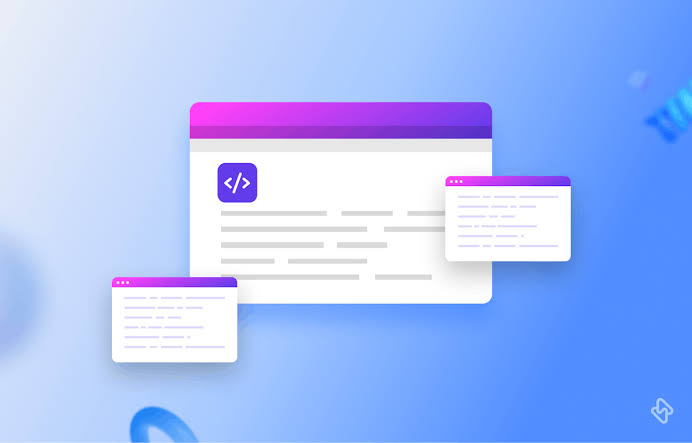
- Define the purpose of the code.
- List prerequisites and dependencies.
- Provide installation or setup steps.
- Write clear instructions on how to use the code.
- Document each function and class with inputs, outputs, and errors.
- Include examples or sample code snippets.
- Explain the workflow or logic flow of the code.
- Add troubleshooting steps for common issues.
- Link to related resources or references.
- Review the documentation for accuracy and clarity.
Example
/**
* This class represents a generic Animal.
*/
class Animal {
protected String name;
/**
* Constructor to initialize the animal's name.
* @param name Name of the animal.
*/
public Animal(String name) {
this.name = name;
}
/**
* Method to make the animal speak (generic behavior).
*/
public void makeSound() {
System.out.println(name + " makes a sound.");
}
}
/**
* This class represents a Dog, which is a subclass of Animal.
*/
class Dog extends Animal {
/**
* Constructor to initialize the dog's name.
* @param name Name of the dog.
*/
public Dog(String name) {
super(name);
}
/**
* Overriding the makeSound method to provide a dog-specific sound.
*/
@Override
public void makeSound() {
System.out.println(name + " barks.");
}
/**
* Method to make the dog fetch an item.
*/
public void fetch() {
System.out.println(name + " is fetching the ball!");
}
}
/**
* Main class to demonstrate inheritance in Java.
*/
public class InheritanceExample {
public static void main(String[] args) {
// Creating an Animal object
Animal genericAnimal = new Animal("Generic Animal");
genericAnimal.makeSound(); // Output: Generic Animal makes a sound.
// Creating a Dog object
Dog myDog = new Dog("Buddy");
myDog.makeSound(); // Output: Buddy barks.
myDog.fetch(); // Output: Buddy is fetching the ball!
}
}
Note: The text inside /* */ and after // is the documentation.